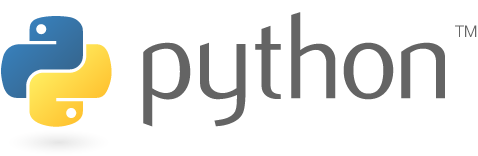
It is hard to recommend Python in production
I started writing in the 2010s when Python 2 was going to be deprecated and Python 3 was too early to support. Python might have died there and then but was picked up by the data science and machine learning community, so, it survived. Running Python in production comes with various gotchas though. Python is resource-intensive Let’s consider a simple Docker image containing “Hello World”. Dockerfile 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 # Build: docker buildx build -t python-fastapi -f Dockerfile_python . # Size: docker image inspect python-fastapi --format='{{.Size}}' | numfmt --to=iec-i # Run: docker run -it --rm --cpus=1 --memory=100m -p 8000:8000 python-fastapi FROM python:3.12-slim AS base WORKDIR /app RUN pip3 install --no-cache-dir fastapi==0.115.11 uvicorn==0.34.0 SHELL ["/bin/bash", "-c"] RUN echo -e "\ from fastapi import FastAPI\n\ app = FastAPI()\n\ @app.get('/')\n\ async def root():\n\ return {'message': 'Hello World'}\ " > /app/web_server.py ENTRYPOINT ["uvicorn", "web_server:app", "--host=0.0.0.0", "--port=8000", \ "--workers=4", "--limit-concurrency=32"] And a similar web server in Go. ...