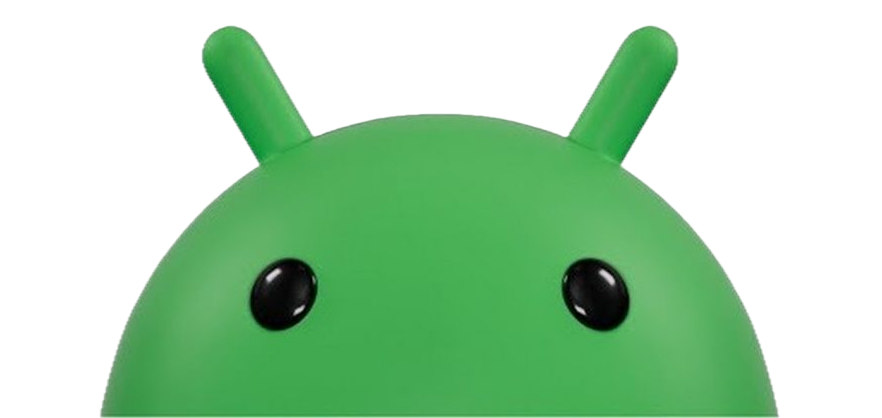
Troubleshooting Android Emulator: Process finished with exit code 1
Emulator: Process finished with exit code 1 You opened AVD Manager in Android Studio and tried to start an AVD, you got “Emulator: Process finished with exit code 1”. Following are the steps to debug this Find out the name of the emulator. Click the down arrow 🔽 which is to the right of play arrow ▶️, to find out the name of the AVD. Let’s say the name is “Nexus_5X_API_28_x86”. ...