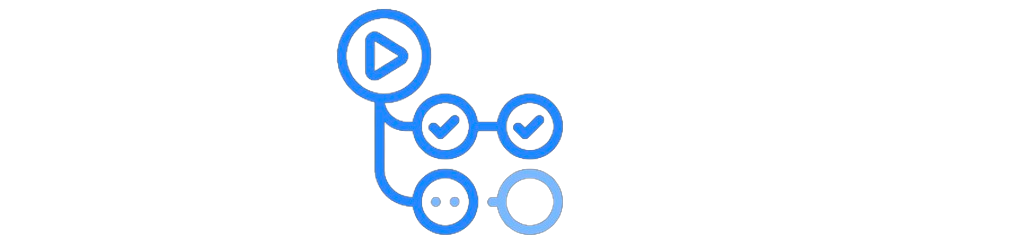
Keep your dotfiles bug-free with Continuous Integration
Ultimate guide to setting up and maintaining CI of your dotfiles
Ultimate guide to setting up and maintaining CI of your dotfiles
Update: As of Mar 2022, I recommend everyone to use GitHub Actions I maintain a somewhat popular Android developer tool ( adb-enhanced). The tool is written in Python, supporting both Python 2 and 3. Testing the tool requires both Python runtime as well a running Android emulator. I, initially, used Travis CI for setting up continuous testing of this tool. Later, I felt that Travis CI was too slow and when I came across Circle CI, I decided to give it a try. As of now, both Travis and Circle CI are used for testing. Here is what I learned from my experience.
Lectures Introduction Creating a Digital currency Bitcoin Overview Bitcoin Blockchain Bitcoin Mining Bitcoin Miner interactions and Game Theory Cryptocurrencies: Community, Economics, and Politics Alternative Consensus Wallet & Anonymity Anonymity on Blockchain Altcoins Ethereum Ethereum Ethereum Governance Bitcoin Side-chains (guest talk) Bitcoin Payment channel Guest talk on Legal by Ben Lawsky - does not seem worthy of transcribing Advanced Topics - Quantum Computing, Threshold Signatures, and storing secret state on public chains Advanced Topics - Smart property, publicly verifiable randomness, and prediction markets Guest talk by Adam Ludwin (CEO, chain.com) - does not seem worthy of transcribing The notes are based on the 2016 version of the course CS251 ...
Lecture 19: Advanced Topics Topic 1: Smart Property Manage ownership of some property like stocks on the blockchain. Colored coins allow arbitrary properties on Smart Contract. Similar to Namecoin, there cannot be a light node/SPV for this. Another example is rental, car’s ownership goes from Alice to Carol in a 2-of-2 transaction from Alice to Carol and locked transaction to return the car’s possession after a fixed time. One still has to trust the car’s hardware and manufacturer. ...
Lecture 18: Advanced Topics Three topics are chosen by students (another three for the next lecture) Topic 1: Quantum Computing An electron has two states top and bottom spins, represented as |1> and |0>. An electron is in a superposition of those two states with wave functions Ψ0 and Ψ1, so, the combined wave function is Ψ = Ψ0. |0> + Ψ1. |1> with |Ψ0|2 + Ψ12 = 1. |Ψ0|2 is the proability of seeing state |0> and Ψ12 is the probability of seeing state |1> respectively. Thus, Ψ = [Ψ0, Ψ1] is the overall state matrix. The state evolves using a 2X2 Hamiltonian matrix H, such that, second-degree norm won’t change, || H.V || = || V ||. This ensures |Ψ0|2 + Ψ12 = 1 always holds. ...
Lecture 16: Bitcoin payment channel Visa ~ 10, 000 transactions per second Bitcoin ~ 3 transactions per second => 60 GB of blockchain data per year Waiting for 6 blocks ~ 60 mins is a huge wait for Bitcoin. Therefore, tipping or having an ongoing channel of payments on the blockchain is hard. Payment channels help with that. Funding channel - unidirectional payment channels Alice is planning to pay Bob. ...
Lecture 15: Bitcoin guest talk (Greg Maxwell & Pieter Wuille - Blockstream) on sidechains Forking does not advance Bitcoin since forks suffer from economic acceptance. UTXO model UTXO model is less intuitive, more private, and smaller persistent storage footprint. UTXO implicitly prevents a replay attack. Ethereum carries nonce around even for empty accounts to prevent replay attacks. Validation not computation Bitcoin addresses are a 160-bit hash of the public key since the public key is unusually long (512-bit). Bitcoin payments can be made to scripts. These scripts are not for computation but spendability conditions. Rather than scripts, a hash of the script is added to the blockchain as a privacy improvement. 10% of Bitcoins are stored using P2SH scripts. MAST is meant to make transactions even smaller. One does not need a Turing-complete language since one only needs to verify and not compute on the blockchain. ...
Lecture 13 - Ethereum Code: ROM (Read-only memory) calldata: arguments There are two types of instructions: Arithmetic including SHA3 and sys operations like create [contract], call [contract], and delegate call, etc. CALL - called code is executed in the context of called contract CALLLOAD - called code is executed in the context of the current contract, msg.sender is calling contract DELEGATECALL - similar to callload except for msg.sender remains unchanged ...
Lecture 14: Ethereum Governance When contracts call other contracts, there are four major parameters, g - gas, v - value, in - in size of inputs, out - out size of outputs. The gas must come from the initial transaction, the ongoing calls to different cannot refuel the gas. By default, all the gas is passed during the contract call and the value passed is 0. A contract can receive money via contract.send(<money_in_wei>) only if it defines a fallback function ...
Recap: alt-coins Bitcoin is a replicated state machine, the system moves within S States with I inputs producing O outputs. For Bitcoin, S is the set of UTXOs. For Namecoin, the state consists (name, value). Ethereum’s goal was to implement this functionality in a general way by building a “consensus computer” expressed in a Turing-complete language. Ethereum State: Great arbitrary storage space, arbitrary code (isolated memory space), and account balance. Inputs: (address, input data) Transition: update storage and change account balance ...